How the async await works in javascript with code samples
-
Table of Contents
- Introduction
- How to Leverage Async/Await to Create Cleaner
- More Readable JavaScript Code
- Debugging Async/Await Code in JavaScript: Tips and Tricks
- Understanding the Benefits of Async/Await in JavaScript: A Comprehensive Guide
- How to Use Async/Await to Make Your JavaScript Code More Efficient
- Exploring the Basics of Async/Await in JavaScript: A Step-by-Step Guide with Code Samples Using Highcharts Maps
- Conclusion
Introduction
Async/Await is a new way to write asynchronous code in JavaScript. It allows you to write asynchronous code that looks and behaves like synchronous code. Async/Await makes it easier to write and read asynchronous code, and it can help you avoid callback hell. Async/Await works by allowing you to write asynchronous code as if it were synchronous code. It does this by using the keyword await, which pauses the execution of the function until the asynchronous operation is complete. For example, let's say you want to make an API call to get some data. With Async/Await, you can write the code like this:
async function getData() {
const response = await fetch('https://example.com/api/data');
const data = await response.json();
return data;
}
This code looks like synchronous code, but it is actually asynchronous. The await keyword pauses the execution of the function until the fetch operation is complete. Once the fetch operation is complete, the response is stored in the response variable and the data is stored in the data variable.
Async/Await makes it easier to write and read asynchronous code, and it can help you avoid callback hell. It is a great tool for writing asynchronous code in JavaScript.
How to Leverage Async/Await to Create Cleaner, More Readable JavaScript Code
Async/Await is a powerful tool for writing cleaner, more readable JavaScript code. Async/Await allows developers to write asynchronous code that looks and behaves like synchronous code. This makes it easier to read and understand, and reduces the amount of code needed to accomplish the same task. Async/Await works by allowing developers to write asynchronous code in a synchronous style. Instead of using callbacks or promises, developers can use the await keyword to pause the execution of a function until a promise is resolved. This allows developers to write code that looks like it is running synchronously, but is actually running asynchronously. Async/Await also makes it easier to handle errors. Instead of having to write complex error-handling code, developers can use the try/catch block to handle errors in a single line of code. This makes it easier to debug and maintain code, as well as making it easier to read. Finally, Async/Await makes it easier to write code that is more modular and reusable. By using the await keyword, developers can break down complex tasks into smaller, more manageable chunks. This makes it easier to reuse code and makes it easier to debug and maintain. Overall, Async/Await is a powerful tool for writing cleaner, more readable JavaScript code. By using the await keyword, developers can write asynchronous code that looks and behaves like synchronous code, making it easier to read and understand. Additionally, Async/Await makes it easier to handle errors and write code that is more modular and reusable.Debugging Async/Await Code in JavaScript: Tips and Tricks
Debugging Async/Await code in JavaScript can be a challenging task. This article provides tips and tricks to help developers debug their code more effectively. When debugging Async/Await code, it is important to understand the underlying concepts. Async/Await is a way of writing asynchronous code that is more readable and easier to debug than traditional callback-based code. Async/Await code is written using the async and await keywords, which allow the code to pause and wait for a promise to resolve before continuing. When debugging Async/Await code, it is important to use the right tools. The most popular debugging tools for JavaScript are the Chrome DevTools and Node.js Inspector. Both of these tools provide a way to step through code line by line and inspect variables. It is also possible to set breakpoints and watch expressions. When debugging Async/Await code, it is important to understand the order in which the code is executed. Async/Await code is executed in a top-down fashion, meaning that the code is executed from the top of the function to the bottom. This means that any code that is written after an await statement will not be executed until the promise has been resolved. It is also important to understand the concept of error handling when debugging Async/Await code. Errors can occur when a promise is rejected or when an exception is thrown. To handle these errors, it is important to use the try/catch block. This will allow the code to catch any errors that occur and handle them appropriately. Finally, it is important to use the right debugging techniques when debugging Async/Await code. It is important to use console.log statements to log out the values of variables and to use the debugger keyword to pause the code and inspect variables. It is also important to use the await keyword to pause the code and wait for a promise to resolve before continuing. By following these tips and tricks, developers can debug their Async/Await code more effectively and ensure that their code is running as expected.Understanding the Benefits of Async/Await in JavaScript: A Comprehensive Guide
Async/Await is a relatively new feature of JavaScript that has revolutionized the way asynchronous code is written. Async/Await allows developers to write asynchronous code in a more synchronous-like style, making it easier to read and debug. In this comprehensive guide, we will explore the benefits of Async/Await and how it can be used to improve the performance of your code. Async/Await is a syntactic sugar for Promises, which are a way of writing asynchronous code in JavaScript. Promises are a powerful tool for writing asynchronous code, but they can be difficult to read and debug. Async/Await makes it easier to read and debug asynchronous code by allowing developers to write code in a more synchronous-like style. One of the main benefits of Async/Await is that it allows developers to write code that is easier to read and debug. Async/Await makes it easier to read and debug asynchronous code by allowing developers to write code in a more synchronous-like style. This makes it easier to understand the flow of the code and identify any potential issues. Another benefit of Async/Await is that it can improve the performance of your code. Async/Await allows developers to write code that is more efficient and can be executed faster. This can lead to improved performance and better user experience. Finally, Async/Await can help developers write more maintainable code. Async/Await makes it easier to write code that is more modular and easier to maintain. This can lead to fewer bugs and a more stable codebase. In conclusion, Async/Await is a powerful tool for writing asynchronous code in JavaScript. It allows developers to write code that is easier to read and debug, can improve the performance of your code, and can help developers write more maintainable code. Async/Await is an invaluable tool for any JavaScript developer and should be taken advantage of whenever possible.How to Use Async/Await to Make Your JavaScript Code More Efficient
Async/Await is a powerful tool for making JavaScript code more efficient. Async/Await allows developers to write asynchronous code that looks and behaves like synchronous code. This makes it easier to read and debug, and can help improve the performance of your code. Async/Await works by allowing developers to write asynchronous code in a synchronous style. This means that instead of writing code that is split up into multiple callbacks, you can write code that looks like it is running in a single thread. This makes it easier to read and debug, and can help improve the performance of your code. To use Async/Await, you must first declare a function as async. This tells the JavaScript engine that the function contains asynchronous code. Then, you can use the await keyword to pause the execution of the function until the asynchronous code has finished running. This allows you to write code that looks like it is running in a single thread, but is actually running asynchronously. Async/Await can help improve the performance of your code by reducing the number of callbacks that need to be executed. This can help reduce the amount of time it takes for your code to run, as well as reduce the amount of memory that is used. Async/Await can also help make your code more readable and easier to debug. By writing code in a synchronous style, it is easier to understand what is happening in the code and to identify any potential issues. Overall, Async/Await is a powerful tool for making JavaScript code more efficient. By allowing developers to write asynchronous code in a synchronous style, it can help improve the performance of your code and make it easier to read and debug.Exploring the Basics of Async/Await in JavaScript: A Step-by-Step Guide with Code Samples Using Highcharts Maps
Async/Await is a relatively new feature of JavaScript that allows developers to write asynchronous code in a more synchronous manner. Asynchronous code is code that runs in the background while the main thread of execution continues. This can be useful for tasks such as making API calls, loading data from a database, or performing calculations. Async/Await is a way of writing asynchronous code that makes it easier to read and understand. It allows developers to write code that looks like it is running synchronously, but is actually running asynchronously. This makes it easier to debug and maintain code. In this article, we will explore the basics of Async/Await in JavaScript. We will look at how to use it with Highcharts Maps, a popular library for creating interactive maps. We will also provide code samples to help you get started. First, let’s look at how Async/Await works. Async/Await is based on the concept of Promises. A Promise is an object that represents the result of an asynchronous operation. It can either be resolved (fulfilled) or rejected. When a Promise is resolved, the code inside the Promise is executed. When using Async/Await, you create an asynchronous function by adding the keyword “async” before the function declaration. Inside the function, you can use the keyword “await” to pause the execution of the function until a Promise is resolved. For example, let’s say you want to make an API call to get some data. You can use the following code to do this:
async function getData() {
const response = await fetch('https://example.com/api/data');
const data = await response.json();
return data;
}
In this example, the function getData()
is an asynchronous function. The keyword “await” is used to pause the execution of the function until the Promise returned by fetch()
is resolved. Once the Promise is resolved, the data is returned from the function.
Now let’s look at how to use Async/Await with Highcharts Maps. Highcharts Maps is a library for creating interactive maps. It allows you to add markers, lines, and other shapes to a map.
To use Async/Await with Highcharts Maps, you need to use the async/await syntax when creating the map. For example, the following code creates a map with a marker:
async function createMap() {
const map = await Highcharts.maps.world();
const marker = await Highcharts.marker(map, {
lat: -25.2744,
lng: 133.7751
});
return map;
}
In this example, the function createMap()
is an asynchronous function. The keyword “await” is used to pause the execution of the function until the Promise returned by Highcharts.maps.world()
is resolved. Once the Promise is resolved, the map is returned from the function. Here is a demo of a working Highcharts Map for you to explore. You can explore the code here Highcharts Map Demo Code
Highcharts Map Demo
This map
Map Demo End
We hope this article has helped you understand the basics of Async/Await in JavaScript. Async/Await is a powerful tool for writing asynchronous code in a more synchronous manner. It makes it easier to debug and maintain code. We encourage you to experiment with Async/Await and Highcharts Maps to create interactive maps.Conclusion
Async/Await is a powerful tool for writing asynchronous code in JavaScript. It allows us to write asynchronous code that looks and behaves like synchronous code, making it easier to read and debug. Async/Await is built on top of Promises and can be used with existing Promise-based code. Async/Await makes it easier to write asynchronous code that is more readable and maintainable. For example, the following code uses async/await to fetch data from an API and log the response:
async function getData() {
const response = await fetch('https://example.com/data');
const data = await response.json();
console.log(data);
}
In conclusion, Async/Await is a great tool for writing asynchronous code in JavaScript. It makes asynchronous code easier to read and debug, and can be used with existing Promise-based code. Async/Await is a powerful tool for writing asynchronous code in JavaScript and should be considered when writing asynchronous code.Great Hosting at Great Prices.
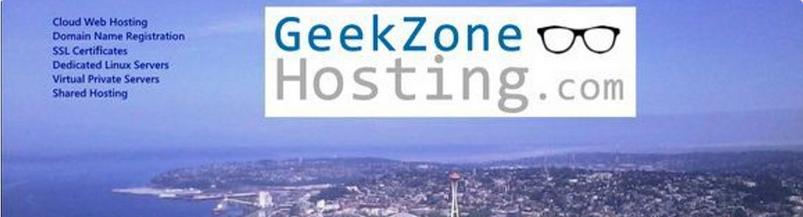
Why Us?
At MTBN.NET, our mission is to provide avant-garde hosting technologies. Our hosting services are equiped with ample data space and bandwidth quotas, domain name registration & transfer options, easy-to-use Domain and Email Managers, multiple website hosting possibilities, as well as charge-free extras, such as a one-click PHP apps installer and a site builder. All accounts can be easily administered through our multilingual Control Panel. A 24-7-365 client care service is available too.
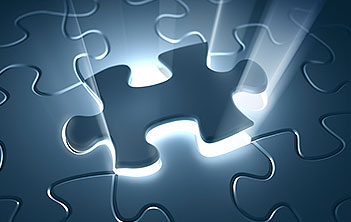
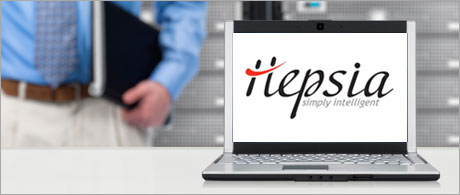
A point-and-click web hosting Control Panel
With your web hosting package, you will get hold of our in-house developed web hosting Control Panel, which will make administering your web presence a breeze. With one mouse click, you will be able to upload a file, to register a domain name or to create a new mailbox. It's that easy! You will also have access to comprehensive real-time traffic statistics.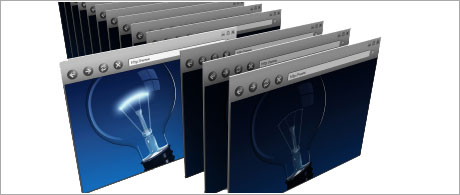
Administer multiple websites
With us, you will be able to manage multiple websites and electronic mail accounts from a single location - our in-house developed hosting Control Panel. You will be able to register, transfer and renew multiple domain names at once, and to manage their settings via an easy-to-handle user interface.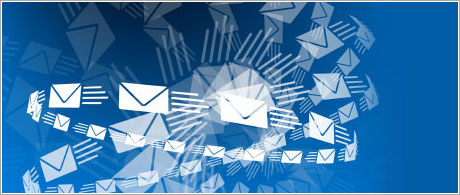
An all-in-one mail management solution
Our all-encompassing Email Manager permits you to set up multiple e-mail accounts and to exercise total command over their settings. You can forward mails, create autoresponder messages and mailing lists and much more. Your mailboxes will be defended against spam electronic mails and malicious software. You can administer your e-mailbox accounts via a webmail client as well.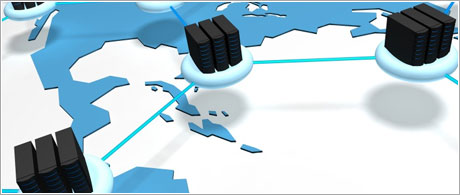
A cloud web hosting platform
We have built a well-balanced hosting platform whereby the load is being distributed across a number of hosting servers. In this way, you never face the risk of going offline because of server overload issues and your web sites will be running unproblematically even if we have a problem with any of the hosting servers.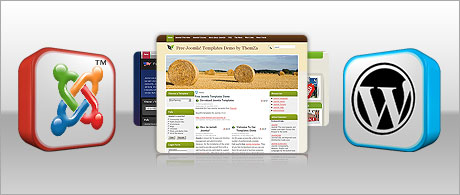
Free-of-cost web design themes
We offer a rich range of free Joomla and WordPress website templates that will help you launch your new site with just a couple of mouse clicks. The Joomla website skins are ideal for all types of dynamic web sites and even e-commerce portals, whereas with the WordPress website themes you can set up your new professional-looking weblog from scratch very quickly.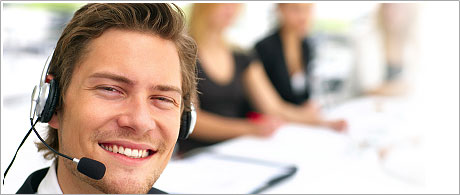
A round-the-clock client care service
We are here 24-7 to respond to your questions and to provide quick assistance whenever you need it. You can reach us by phone or via live chat for any pre-sale or general questions, or via e-mail or through the Control Panel-incorporated ticketing system for any technical issues. Never hesitate to touch base with us at any time.Get 10,000 free PLR-Private Lable Rights articles to use on your blog. Order your Domain and Hosting and then Email us your domain name certificate.
We will send you back your 10,000 free Private Lable Rights articles you can use on your blog after you process them at PLRImporter.Com
Looking for a easy way to set up your online store? Check out our favorite way-Set up Your Online Store